C Sharp Programming Language
Is Csharp easier than C++? Shan Trainings
C# programming language is used to develop application that run in .NET framework candidates learn to develop windows applications, web applications and games using programming language C# and with this coding skill you can easily earn a lot of money by joining any organization as a C# developer we may each and every topic in this programming course from basic to pro level to increase the programming skills of students after FA by working on projects and assignment you can learn to build high-quality and powerful applications in C-Sharp under the guidance of programming experts. Here is the advantages of C# which you may learn;
- C# is the object-oriented
- It is the part of the C-Family of Languages
- High Scalability
- Extensive Libraries
- C# has large community
- It is easy to learn
- Allows developer to use .NET libraries
- Better Code quality
- Faster development
Genuine Tech is the best Computer College for programming courses in which boys and girls can learn each topic individually for better learning and knowledge gaining upon the completion of our programming trainings we may provide certificate to each candidate which may help to get job in future and they become expert in their professions like in this C# programming training we may include each and every topic from basic to advance level in which candidates can learn the basic syntax of C# with advance features.
It is the C-Family language and have object-oriented programming concepts in which students can learn inheritance, polymorphism and many more. C# is the top programming language in world which you can online as well specially design for students who can not join us physically can avail the opportunity to learn this high in demand computer programming course and become programming expert like a professional.
Read from pluralsight.com to get more information about C# programming.
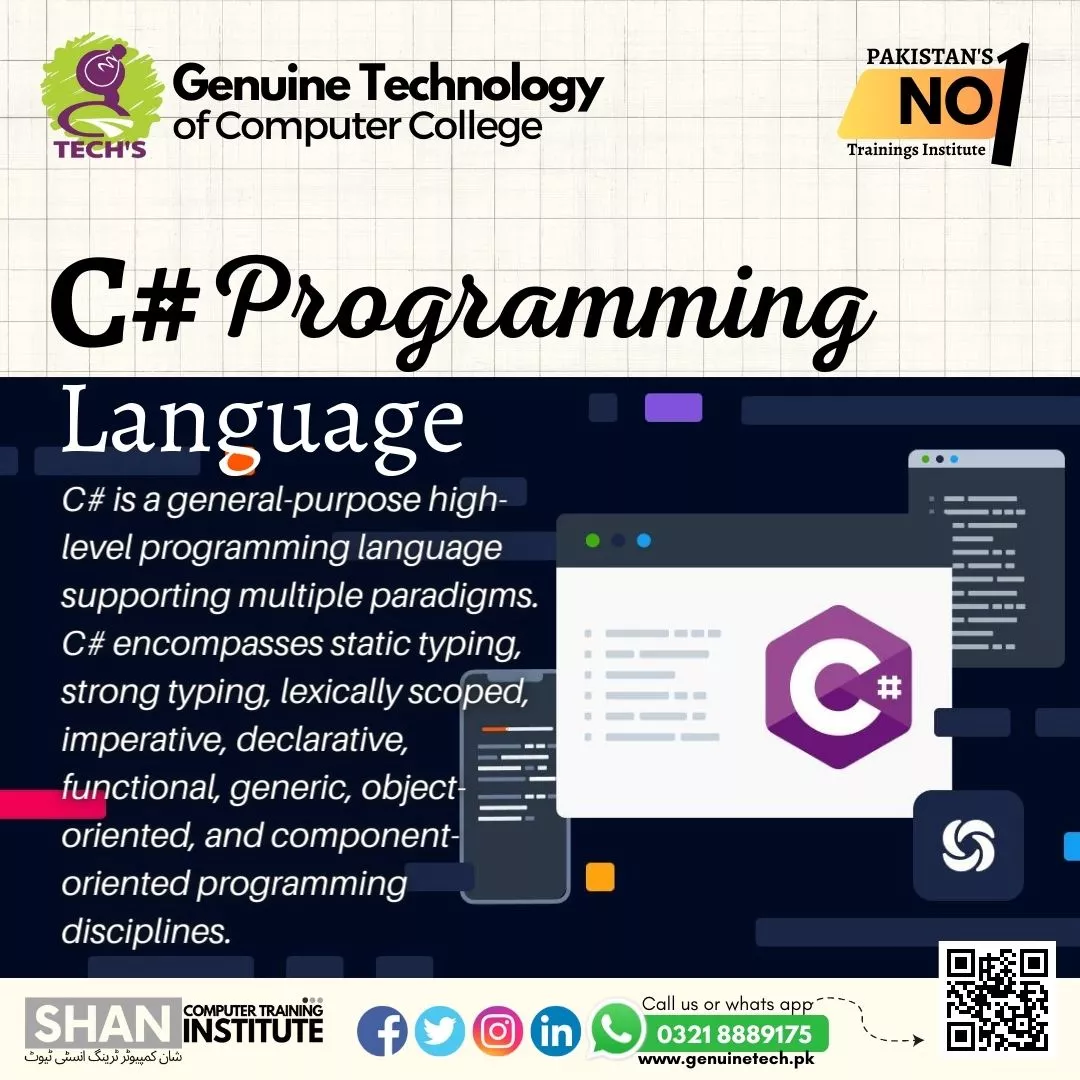
If you want to get further details and update about computer trainings course don't hesitate direct whatsApp chat, call or visit and follow Facebook page, Instagram and get more information LinkedIn accounts