Advance PHP with Laravel Framework
Can I use Laravel with PHP? - shan trainings
Advance PHP with Laravel Framework training by Genuine Tech. Learn to create top notch websites under the guidance of experts and design web apps in the Laravel PHP Framework which is in high demand and web development is one of the best computer courses to learn which is beneficial for your career and to enhance your computer skills. Here in this advance php with laravel course you can learn;
- Introduction to Laravel
- Object-Oriented Programming (OOP)
- Composer
- Routing
- Middleware
- Controllers
- Views
- Models
- Migrations
- Authentication
- Authorization
- Eloquent ORM
- Query Builder
- Blade Templating Engine
- Validation
In this Advance PHP with Laravel Course you learn about the open-source PHP web framework designed for building web applications with elegant syntax and features. Understanding the OOP concepts is important in Laravel development. Learn about the Composer, Routing and Middleware which can be used in handling dependencies within your projects. Learn about the complete MVC architecture Model, View and Controllers. You can learn about the Migrations and Authentication in Laravel which allow you to make changes to your database and Laravel provide an easy authentication system which may allow to authenticate users and control access to your application.
Advance PHP with Laravel Framework Techniques and Features
Genuine Tech is one of the Top Computer College in Lahore in which you learn advance php with laravel framework techniques and features like Eloquent ORM which may allow you to work with databases using objects, Query Builder in Laravel which may provides a simple query builder for creating database queries, Blade templating engine in laravel which may allow you to create reuseable views. Laravel may provide a built-in validation system that allows you to validate user input.
You can learn the advance php with laravel computer course in Lahore in which you can learn the advance features and techniques which may include Error Handling, Artisan CLI, Service Container, Events and Listeners, Queues, Broadcasting, Notifications, API Development, Testing, Caching, File Storage, Localization, Task Scheduling, Socialite and Deployment and you may mastering the advance php with laravel framework.
Is Laravel the best PHP framework
With it's elegent features and capabilities for web designing and development projects Laravel is the perfect PHP framework for developing web applications recommend or prefer by most of the developers. Candidates can learn expert PHP development with Laravel for professionals and enhance your skills and knowledge you can use Laravel with PHP and create complex web applications with ease.
What is the best way to learn Laravel
You may enroll in Genuine Tech Laravel training course in which you may learn laravel by practicing coding on real-world web development projects as a beginner we may provide you the small web-based projects to practise and with the passage of time you can create your own website design & development using laravel web programming this may increased your skills and knowledge as you clear the pojects or task on daily basis it may enhance laravel developer skill and you become proficient expert larave developer.
This Advanced PHP with Laravel Course teaches you about Laravel, an open-source PHP web framework built for creating online applications with attractive syntax and capabilities. Understanding the OOP fundamentals is critical in Laravel programming. Learn about Composer, Routing, and Middleware, which may help you manage dependencies in your applications. Learn about the MVC architecture's Model, View, and Controllers. You may learn about Migrations and Authentication in Laravel, which allow you to make changes to your database. Laravel also provides a straightforward authentication system that allows you to authenticate users and manage access to your application.
You can take an advanced php with laravel computer course in Lahore and learn advanced features and techniques such as error handling, artisan CLI, service container, events and listeners, queues, broadcasting, notifications, API development, testing, caching, file storage, localization, task scheduling, socialite, and deployment. You can also master the advanced php with laravel framework.
Expert Tips For Laravel PHP Development
In this topic you may learn pro-level laravel php coding techniques through which you can develop dynamic web interfaces and create server-side logics for database management and coding scalability you can get advance tips and tricks for creating routes and optimize the website performance under the guidance of expert full stack laravel developers this is one of the best laravel training course in Lahore in which you can enroll as a beginner to gain knowledge or as a professional to enhance your laravel developer skills.
First you can clear the basic PHP laravel development concepts and features along with object-oriented programming then create small website projects once the completion of this training you can develope your own portfolio and apply for employment in the job market as a laravel developer. Top courses in Laravel and PHP may unlock your career opportunities and skill enhancement in tech industries and laravel is always in demand in website development.
Mastering PHP Advanced Concepts with Laravel
In this advance php with laravel training you may mastering php advanced concepts with laravel you can learn tips and techniques for laravel with php development for creating websites and learn object-oriented programming which may include Inheritance, polymorphism, abstracton and many more.
Working with SQL languages you can develop unique database designs and queries through which you can manage the structure of your website database and integrate it with web application projects with ease and learn the unique feature of php programming like security tools and data handling techniques, vast library with functions and many more upon the completion of this laravel development training you may become full stack php laravel developer.
Why Choose Genuine Tech?
Genuine Tech is the professional web design company in Lahore here you can learn the training of custom website development individually for Males and Females they may also provide website redesign services in Pakistan for clients. In this way they may provide multiple services related to education and development of our country. Here you can learn Frontend web development, Backend web development, website maintenance services, website optimization services or can start working online or physically as well in all over the world.
By visiting builtin.com you can learn benefits of laravel.
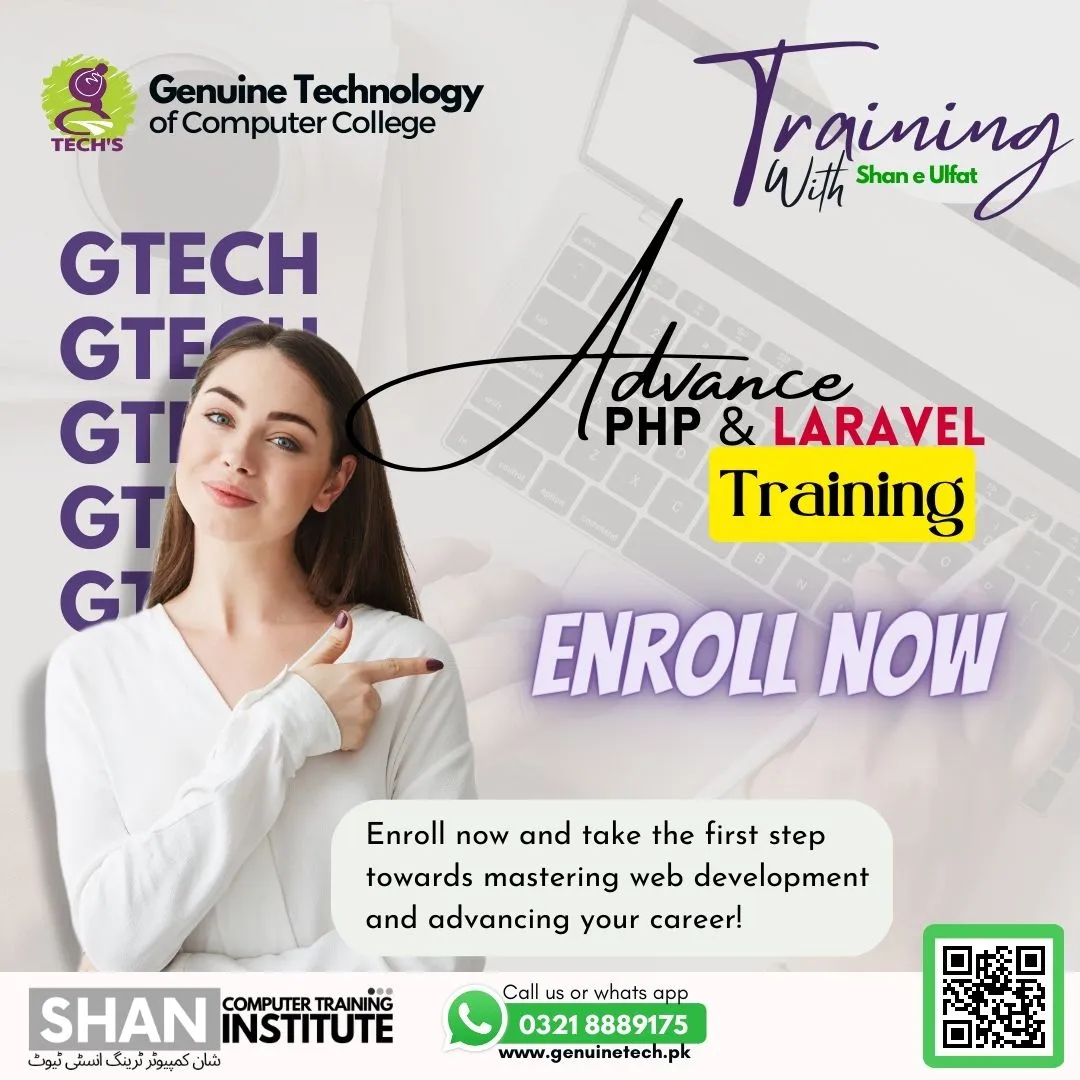
If you want to get further details and update about computer trainings course don't hesitate direct whatsApp chat, call or visit and follow Facebook page, Instagram and get more information LinkedIn accounts