Object Oriented Programming Courses
Learn Object Oriented Programming Trainings Course for Beginners
In the Programming field we know that Object-Oriented Programming is very important because it is the computer programming model which is used in organizing the software design around data and objects and it is totally based on the concept of objects which can contain data and code. As a programmer whether you can choose high level programming language or a basic programming language like C++ you can use the object-oriented programming in it in order to create software development or an application development. Most of the companies job description may include the core concepts and the pillars of object-oriented programming OOP.
It is important in any programming language to learn OOP and its fundamentals in order to observe these requirement for the candidates College of Genuine Technology and Shan Computer Trainings Institute is providing the programming fundamentals course in which we include the object-oriented programming in C++ for youth development in the programming field in this programming fundamental course you can learn;
- C++
- OOP
- Class
- Objects
- Encapsulation
- Abstraction
- Polymorphism
- Inheritance
- Dynamic Binding
- Message Passing
Programming Fundamentals and its Process
In order to start programming in any language you may know the basic principles and the fundamentals of programming which may include Variable declaration, Control structures, Data structures, object-oriented programming, Debugging and the Programming tools. In the programming process you need to work with one approach to solve the problems those things are Identify the problem, design a Solution, Write the program which you can usually say programming and Check the Solution. Here are the 7 fundamental elements of programming;
- Data: constants, variables.
- Input: reading of values from input device.
- Output: put in writing of information to any output device.
- Operations: comparing values, assigning values.
- Conditions / Selections: If-Then-Else, Case, Switches.
- Loops / Iterations: While-Do, Repeat Until, For-Do.
- Subroutines / Modules: Functions, Procedures.
Object Oriented Programming in C++
OOP stands for object-oriented programming and in this programming all is about to deal or create the objects that contain both data and functions in this programming fundamentals course we introduce you the OOP, Difference between OOP and Procedural programming and Understanding classes and objects In object-oriented programming we can divides the program into small parts and refers to them as objects while in procedural programming we can divide the program in to small parts and refers to them as functions. Object-oriented programming few advantages over procedural programming;
- OOP is faster and easier to execute.
- It provides a clean and proficient structure for the programs.
- It helps to keep the C++ code DRY “Don’t Repeat Yourself”, and makes the code easier to maintain,
- update and debug.
- With OOP you can create reusable applications with less code and in shorter time.
In C++ object-oriented programming language use objects to model real-world problems and an object has two properties attributes and behavior. For example, a car can be an abject it has the following attributes brand, model, size and mileage and many more and has behavior like driving, acceleration, parking and many more.
C++ Classes and Objects
In programming languages C++ is basic language and easy to learn Class in C++ is the building block that leads to object-oriented programming. It is the user defined data type which have its own data members and member functions, which can access and used by the programmer by creating an instance of that class. In simple definition you can say a class is a blueprint for an object or in order words you can assume that the class as a sketch of a senate which contain all the details about the floors, doors and windows based on this description we can construct the senate. Senate is basically the object here in programming fundamentals by using C++ programming language you can working in classes and objects by using the OOP concepts.
A class Is defined using the keyword class in C++ followed by the name of the class and the body of the class is defined inside the curly brackets and finishes by a semicolon at the end of the syntax. For example, Consider the Class of Students all of them having different names, age and color but all of them in the class can learn the same computer course under the teaching of same teacher so, Students is the class, and computer course and teacher are their properties.
- A Class is a user or a programmer define data type that has data members and member functions.
- Data members are the data variables and member functions used to operate these variables together it may define the properties and behavior of the objects in a Class.
An object is an instance of a Class in the C++ object-oriented programming when we define a class there is no memory allocated but when an is created than memory is allocated. An object is created to use the data and access functions which is defined in the class.
Why we give semicolons at the end of class?
According to the programmers or many people say that it’s a basic syntax that we should give a semicolon at the end of the class as it is the rule defines in cpp programming. But the reason why we used the semicolon is that the compiler checks that whether we want to create an instance of the class at the end of it. Because we can create the instance of a class at the end.
Data Members and Member Functions in C++
In Programming fundamentals the variables with data types like integer, float, char, string which is declared in any class refers to as a Data member and the Functions which is declared in the private or in the public section of the class are known as Member functions. There are two types of data members and member function in C++;
- Private Members: This is the type of members which are declared in the private section of the class using private access modifier and they only accessible within the same class in which they are declared.
- Public Members: These members are declared in the public section of the class using public access modifier refers to as public members and can be access within the class and outside of the class of the class by using the object name of the class in which they are declared.
Basic Principles of C++ Object-Oriented Programming
In this programming fundamentals course we can discuss about the basic principles of OOP and this is also known as the 4 pillars of OOP which is Encapsulation, Abstraction, Inheritance and Polymorphism with out these concepts you cannot understand or complete the programming fundamental of object-oriented programming in C++. Here are the principles of OOP;
Encapsulation | It may tie together the related data and functions which is present within a single entity. |
---|---|
Abstraction | This may show only the essential attributes of a class and hiding the unnecessary and technical details from the user |
Inheritance | It may inherit the features of the existing class within a new class without creating the change in the existing class. |
Polymorphism | Polymorphism means many form and in programming it has the ability of the same entity to behave or act differently in different places. |
Encapsulation in OOP
Encapsulation is defined as storing or wrapping up data under a single unit In C++ object-oriented programming encapsulation is used to binding the data and the functions that operate together. In order to understand the encapsulation in the real world you can see a company which have many different departments like the development, finance and sale etc. Each department can keep the record according to their department So, if one department wants the record of another department so they cannot access the data directly from another department it is not allowed they need permission from the other department and then further proceed this is the same in encapsulation which hide data from one department to another department.
Abstraction in OOP
Abstraction is the most important features of object-oriented programming in which the user can show the necessary information and hiding the complex detail of the program implementation and execution. In programming we can done abstraction by these 2 methods;
- Abstraction using Class in C++: In C++ programming we can use the concept of abstraction by using the classes we know that in class there is a data members and functions is present so the class is decide which data member will be shown to the peoples and which is not.
- Abstraction in Header files in C++: We can do abstraction in header files in C++ through which we can hide the algorithm which is performing the tasks and show only the result to the users.
Inheritance in C++
Inheritance is a method through which one class can inherits the properties from its parent class. In programming fundamental, you can say a derived class can inherits the characteristic from its base class which is also called super class. Inheritance supports the concept of reusability like when we want to create a new class and we already have a class which have some code which is needed so we can create our new class from the base class. Here are the 5 different types of inheritance in C++;
- Single Inheritance: A type of inheritance in which a class derives from one base class only.
- Multiple Inheritance: In this type of inheritance a class is derived from more than one class.
- Multilevel Inheritance: In which a class is derived from another derived class.
- Hierarchical Inheritance: In hierarchical inheritance more than one class create from a single base class.
- Hybrid Inheritance: It is the combination of more than one type of inheritance.
Polymorphism in C++:
Polymorphism means many form and polymorphism in C++ means the same function or an object behaves differently in different layout. Like the “+” operator in Cpp programming can perform two different layouts when it is used in integer like in number so they act as to sum these numbers and on the other hand same operator is used for the concatenation when it comes to strings. Here is types of polymorphism in C++;
- Compile Time Polymorphism: In which the function is called at the time of program compilation.
- Runtime Polymorphism: In which the function is called at the time of program execution.
Dynamic Binding
C++ programming has virtual functions to support Dynamic binding in which the program is executed in getting response to the function call which is decided at runtime. It allows us flexibility by calling a single function to handle different objects.
Static Binding
In C++ programming the binding which is created at compile time is known as static binding and in C++ there is a default route is present for static binding. And it is resolved by the compiler at compile time. Here are the advantages of Static binding;
- It is faster than dynamic binding.
- It optimize the speed of program to run quickly and efficiently.
Advanced Concepts in OOP and C++
In advanced concepts of OOP and C++ programming fundamentals course we may include File handling, Constructor and Destructor, Function Overloading, Function Overriding, Virtual Function and the Pure Virtual Function. Genuine Tech is the best programming training institute which may provide these advanced concepts training in programming fundamental course and the student can learn from beginner level to advance level programming and its concept. Here is the Advanced concepts in OOP and C++;
- File Handling.
- Constructor.
- Destructor.
- Function Overloading.
- Function Overriding.
- Virtual Function.
- Pure Virtual Function.
What are the advantages of OOPs concepts?
Here are the few advantages of OOPs concepts;
- Troubleshooting is easier with the OOP language.
- Code Reusability.
- Productivity.
- Data Redundancy.
- Code Flexibility.
- Solving Problems.
- Security.
What the difference between OOP and procedural programming?
In object-oriented programming you may organize your code by creating objects while in procedural programming you may use a detailed list of instruction to tell the computer what to do step by step.
What is constructor in OOP C++?
It is a special member function in C++ programming which have the same name as that of its class and used to initialize some valid values to the data members of an object.
Conclusion
At conclusion in Genuine Tech you can learn this best programming course which is based on the real life projects and task learning you may apply OOP and C++ skills to real projects and developing a software application or a game presenting the project and getting feedback. This programming fundamentals C++ and OOP course can be the best for your career development and you can become an expert in programming like a professional and after this start your career further in the web development in order to become the full stack web developer according to your interest.
To get more info about Object oriented programming click here.
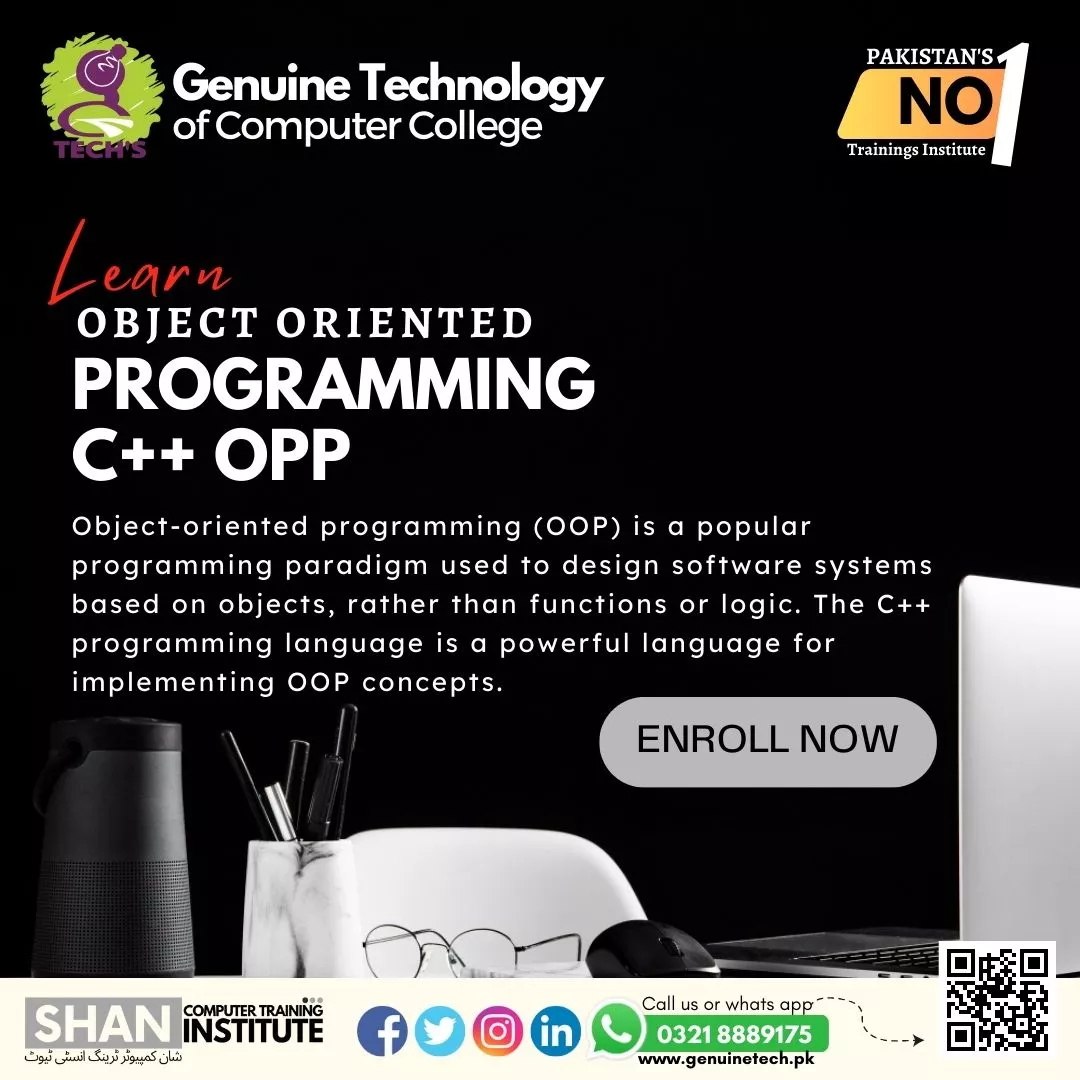
If you want to get further details and update about computer trainings course don't hesitate direct whatsApp chat, call or visit and follow Facebook page, Instagram and get more information LinkedIn accounts